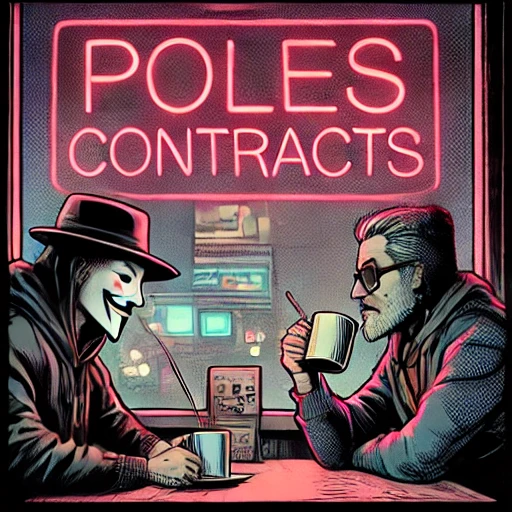
Automating Remote File Synchronization and Permission Management with a Bash Script
Created by: laczi.ostr
Automating Remote File Synchronization and Permission Management with a Bash Script
Subtitle: A simple guide to automating file synchronization and managing permissions on a remote server using SSH and rsync.
Introduction
As modern web development evolves, developers often work with remote servers to deploy, update, and maintain their websites or applications. Keeping local and remote directories synchronized while maintaining correct file and folder permissions is critical for security and functionality. Automating these tasks not only saves time but also minimizes the risk of human error. One powerful tool to achieve this is a Bash script that leverages SSH and rsync.
This article introduces a Bash script that automates the synchronization of files between a local directory and a remote server directory, along with setting appropriate permissions for files and directories. We will break down the script step-by-step, covering how it works, how to set it up, and the significance of each command. By the end of this guide, you’ll be able to manage file synchronization and permissions for your remote server in a secure and automated way.
Main Content
The script provided is designed to help developers efficiently sync their local files to a remote server and apply the necessary permissions. It uses several key tools: SSH for secure remote connections and rsync for synchronizing files between local and remote directories. Below is a breakdown of how the script functions.
1. Script Breakdown
Here’s a look at what each part of the script does:
# Variables
KEY_PATH="your_private_key.pem" # Path to your private key file
REMOTE_USER="your_remote_user" # Remote server user
REMOTE_HOST="your_remote_host" # Remote server address
REMOTE_DIR="/var/www/html/your_remote_dir" # Remote directory to sync and apply permissions
LOCAL_DIR="/path/to/your/local_directory" # Local directory to sync from
# Clear the destination folder on the remote server
echo "Clearing old files from the destination folder on the remote server..."
ssh -i "$KEY_PATH" "$REMOTE_USER@$REMOTE_HOST" "sudo rm -rf $REMOTE_DIR/*"
if [ $? -eq 0 ]; then
echo "Old files cleared from the destination folder."
else
echo "Error: Failed to clear the destination folder."
exit 1
fi
# Sync folders between source and destination with verbose output
echo "Starting folder synchronization..."
rsync -av -e "ssh -i $KEY_PATH" "$LOCAL_DIR/" "$REMOTE_USER@$REMOTE_HOST:$REMOTE_DIR"
if [ $? -eq 0 ]; then
echo "Folder synchronization completed."
else
echo "Error: Folder synchronization failed."
exit 1
fi
# Set folder permissions to 755 for all directories (including subdirectories) on the remote server
echo "Setting directory permissions to 755..."
ssh -i "$KEY_PATH" "$REMOTE_USER@$REMOTE_HOST" "find $REMOTE_DIR -type d -exec chmod 755 {} \; -print"
if [ $? -eq 0 ]; then
echo "Directory permissions set."
else
echo "Error: Failed to set directory permissions."
exit 1
fi
# Set file permissions to 644 for all files (including files in subdirectories) on the remote server
echo "Setting file permissions to 644..."
ssh -i "$KEY_PATH" "$REMOTE_USER@$REMOTE_HOST" "find $REMOTE_DIR -type f -exec chmod 644 {} \; -print"
if [ $? -eq 0 ]; then
echo "File permissions set."
else
echo "Error: Failed to set file permissions."
exit 1
fi
# Print completion message
echo "Folder synchronization and permission setting completed."
This Bash script helps automate several steps in the process of syncing files to a remote server and setting the necessary permissions. Each section is broken down into easily understandable components.
2. Clearing Old Files from the Remote Server
This SSH command connects to the remote server using the specified private key, user, and host. It then removes all files from the specified remote directory, ensuring the folder is empty before new files are synced.
3. Syncing Local Files to the Remote Server
This command uses rsync to synchronize the local directory with the remote directory. It transfers files securely over SSH, preserving permissions and file structures. The -a flag ensures that all files and directories are copied recursively, and the -v flag enables verbose output to display the details of the sync process.
4. Setting Permissions for Directories and Files
After the files have been synced, it’s important to apply the correct permissions to ensure proper access control on the server:
This command sets the permission for all directories to 755. This means the owner has full read, write, and execute permissions, while everyone else has read and execute permissions only.
This command sets file permissions to 644. The owner can read and write the files, while everyone else can only read them.
5. Final Completion Message
The final echo command confirms that the script has completed its task.
How to Install and Use the Script
To use this script, follow these steps:
1. Install Necessary Tools
Before running the script, make sure you have the following tools installed:
- OpenSSH: This should already be installed on most Linux distributions. If not, you can install it using
sudo apt-get install openssh-client
. - rsync: To install rsync, use
sudo apt-get install rsync
.
2. Set Up SSH Keys
If you don’t have an SSH key pair, create one with the command ssh-keygen
and copy the public key to the remote server using ssh-copy-id
. Ensure that you have proper access to the remote server using your private key file.
3. Customize the Script
Update the script with your own variables such as the KEY_PATH
, REMOTE_USER
, REMOTE_HOST
, and directories.
4. Run the Script
Once everything is set up, run the script by executing the command:
This will start the synchronization process and apply the correct permissions automatically.
Conclusion
By using this script, developers can automate file synchronization between their local machines and remote servers, while ensuring that proper permissions are applied to both files and directories. This not only streamlines the deployment process but also reduces the chances of permission-related issues. As web development becomes more complex, having an automated workflow like this one is an essential part of efficient server management.
References
- Official rsync Documentation: https://linux.die.net/man/1/rsync
- OpenSSH Documentation: https://www.openssh.com/manual.html