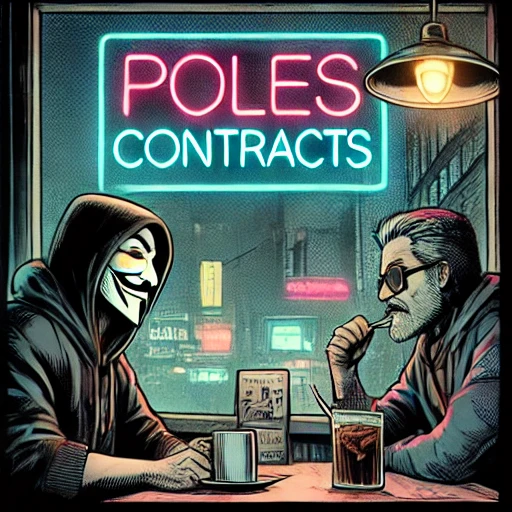
Generating Wi-Fi Maps from CSV Data Using Python and Wigle.
Created by: laczi.ostr
Generating Wi-Fi Maps from CSV Data Using Python and Wigle
Wi-Fi network mapping has become an essential tool for network security professionals, penetration testers, and researchers seeking to visualize the spread and density of wireless networks. The Wigle Wi-Fi database is an extensive platform that collects data on Wi-Fi networks worldwide, and leveraging this data for geographical visualization can offer valuable insights. In this guide, we will explore how to generate a map from CSV data obtained through Wigle Wi-Fi using Python. This step-by-step guide will explain how to extract, clean, and map Wi-Fi data efficiently.
Introduction
In today's increasingly connected world, wireless networks are ubiquitous. Whether you are at a coffee shop, in a library, or walking through a city center, you are likely within range of multiple Wi-Fi networks. With this proliferation of wireless networks comes the need for tools that can help individuals visualize and analyze them. Wigle Wi-Fi is one such platform, which crowdsources Wi-Fi network data from users who scan for and upload network information. This project aims to provide an efficient way to generate maps from the CSV data that Wigle Wi-Fi provides, making network visualization more accessible to users.
For those unfamiliar, Wigle Wi-Fi allows users to collect data about Wi-Fi networks and store it in CSV files, which contain details about SSIDs, GPS coordinates, encryption types, and more. By converting this raw data into a map, users can visualize the location and density of wireless networks, which can help with tasks such as site surveying, penetration testing, and even urban network planning.
In this article, we will walk you through the process of generating a Wi-Fi map from CSV data. This step-by-step guide is ideal for beginners with a basic understanding of Python. We'll use libraries such as `pandas` for data manipulation and `folium` for generating maps, allowing us to plot Wi-Fi data points on an interactive map. By the end of this guide, you'll have a working solution to convert Wigle Wi-Fi data into a visually appealing map that you can interact with.
Steps to Generate a Wi-Fi Map
Step 1: Install Required Libraries
Before you begin, you’ll need to install the necessary Python libraries. Run the following commands in your terminal to install the required libraries:
pip install pandas folium
`pandas` will allow you to load and manipulate CSV data, while `folium` will help generate the actual map. Once these are installed, you're ready to start building your project.
Step 2: Loading and Cleaning the CSV Data
Next, you will load the CSV data from Wigle Wi-Fi using `pandas`. It's important to first inspect the data to understand its structure. The CSV file will typically contain columns such as SSID (network name), BSSID (MAC address), GPS coordinates (latitude, longitude), and encryption type.
import pandas as pd # Load the CSV file df = pd.read_csv('wigle_wifi_data.csv') # Display the first few rows print(df.head())
Take a moment to inspect the data and determine which columns are essential for the map. For this guide, we will focus on the latitude, longitude, and SSID columns. Additionally, you may want to clean the data by removing any duplicate entries or rows with missing GPS coordinates.
# Remove rows with missing GPS coordinates df_cleaned = df.dropna(subset=['lat', 'lon']) # Remove duplicate entries based on BSSID (MAC address) df_cleaned = df_cleaned.drop_duplicates(subset='BSSID')
Step 3: Creating a Map with Folium
Now that we have clean data, we can proceed to generate the map. We'll use the `folium` library to create an interactive map where each Wi-Fi network is marked by its GPS coordinates.
import folium # Initialize a map centered at an average location wifi_map = folium.Map(location=[df_cleaned['lat'].mean(), df_cleaned['lon'].mean()], zoom_start=12) # Add markers for each Wi-Fi network for index, row in df_cleaned.iterrows(): folium.Marker([row['lat'], row['lon']], popup=row['SSID']).add_to(wifi_map) # Save the map as an HTML file wifi_map.save('wifi_map.html')
This code initializes a map centered on the average latitude and longitude from the data. It then iterates over each row in the cleaned dataset, adding a marker for every Wi-Fi network found. The SSID of each network will be displayed when a marker is clicked.
Step 4: Visualizing the Map
Once the map is generated, you can open the `wifi_map.html` file in your web browser to see the result. Each Wi-Fi network will be displayed as a marker on the map, and you can zoom in and out to explore the data further.
Conclusion
Visualizing Wi-Fi networks using data from Wigle Wi-Fi is a powerful way to gain insights into the distribution and density of wireless networks in a given area. By converting CSV data into an interactive map, you can better understand the spread of networks, which is useful for network security assessments, city planning, and general research purposes. This guide provided a simple yet effective method of using Python to manipulate CSV data and generate visual representations using the `pandas` and `folium` libraries. With this tool in hand, you can easily explore Wi-Fi networks and enhance your data analysis capabilities.
References
- Wigle Wi-Fi Project: https://wigle.net/
- Folium Documentation: https://python-visualization.github.io/folium/
- Pandas Documentation: https://pandas.pydata.org/docs/